Introduction
In the realm of data science, Pandas, a versatile and powerful Python library, has emerged as a cornerstone for data manipulation and analysis. One fundamental task in working with Pandas DataFrames is accessing and understanding the column names. These names serve as identifiers for each column, organizing the data and enabling us to extract meaningful insights. In this comprehensive guide, we will embark on a journey to unveil the various techniques for obtaining column names in Pandas, empowering you to navigate your data with precision and efficiency.

Image: webframes.org
The Basics: Understanding Column Names
A DataFrame, the workhorse of Pandas, is a tabular data structure consisting of rows and columns. Each column represents a specific variable or attribute, and its corresponding name provides context and identifies its contents. Column names are essential for accessing, manipulating, and understanding the data within the DataFrame, guiding our exploration and analysis.
Method 1: Utilizing the `columns` Attribute
The most straightforward method of retrieving column names in Pandas is through the `columns` attribute. This attribute returns a Python Index object containing the list of column names. By accessing this attribute, we can easily display or work with the column names:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame('Name': ['Alice', 'Bob', 'Carol'], 'Age': [25, 30, 35])
# Get the column names using the columns attribute
column_names = df.columns
# Print the column names
print(column_names)
Method 2: Employing the `keys()` Function
Another approach to obtaining column names is via the `keys()` function. This function retrieves the keys of the DataFrame, which correspond to the column names. The `keys()` function works particularly well for accessing both column names and row indices simultaneously, offering a convenient alternative:
# Get the column names using the keys() function
column_names = df.keys()
# Print the column names
print(column_names)
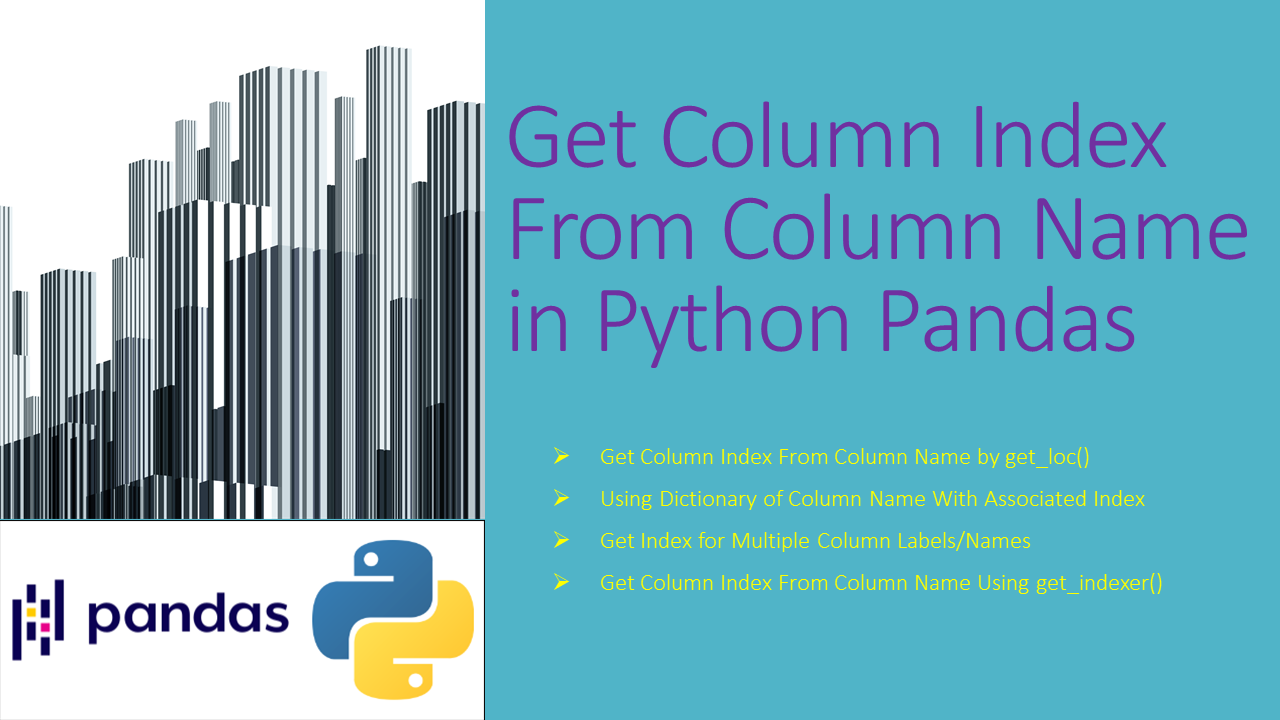
Image: sparkbyexamples.com
Method 3: Leveraging the `values()` Function
While the `values()` function primarily retrieves the values within a DataFrame, we can leverage it to access column names as well. By invoking `values()` on the DataFrame’s columns attribute, we obtain a NumPy array containing the column names:
# Get the column names using the values() function
column_names = df.columns.values
# Print the column names
print(column_names)
Advanced Techniques
In addition to these fundamental methods, Pandas provides advanced techniques for working with column names. These techniques offer greater flexibility and control:
Converting Column Names to Lowercase or Uppercase
# Convert column names to lowercase
df.columns = df.columns.str.lower()
# Convert column names to uppercase
df.columns = df.columns.str.upper()
Renaming Columns
# Rename a single column
df.rename(columns='Old_Name': 'New_Name', inplace=True)
# Rename multiple columns
df.rename(columns='Name': 'Full Name', 'Age': 'Years Old', inplace=True)
Dropping Columns
# Drop a single column
df.drop('Column_Name', axis=1, inplace=True)
# Drop multiple columns
df.drop(['Column_Name1', 'Column_Name2'], axis=1, inplace=True)
How To Get Column Names In Pandas
Conclusion
Mastering the art of obtaining column names in Pandas unlocks a world of possibilities for data analysis and exploration. The techniques outlined in this comprehensive guide provide a solid foundation for understanding and working with DataFrame columns. By leveraging these methods, you can navigate your data with precision, extract meaningful insights, and elevate your data science endeavors to new heights. As you continue your data exploration journey, remember to embrace these techniques and unleash the power of Pandas to unlock the secrets hidden within your data.